In the App, the drawer is very common, so let's see how to use the drawer;
1. Scaffold
The Scaffold is a container that defines a parameter with drawer, As follow:
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
actions: <Widget>[
Padding(
padding: EdgeInsets.only(right: 10.0),
child: IconButton(
onPressed: () {},
icon: Icon(Icons.more_vert),
),
)
],
),
body: body,
floatingActionButton: bottomIcon == null
? null
: FloatingActionButton(
onPressed: () {},
child: Icon(bottomIcon),
),
drawer: this.hasDrawer
? CommonDrawer(
context: context,
title: title,
)
: null,
);
}
2. Define our drawer
Above code, we can see the class CommonDrawer, which is our defining drawer. Let's see the code:
///
/// Created by NieBin on 18-12-17
/// Github: https://github.com/nb312
/// Email: niebin312@gmail.com
///
import "package:flutter/material.dart";
import 'package:flutter_ui_app/const/string_const.dart';
import 'package:flutter_ui_app/const/images_const.dart';
import 'package:flutter_ui_app/const/size_const.dart';
import 'AboutMeTitle.dart';
const ITEMS = [
{
"title": PageConst.profileOneRoute,
"color": Colors.blue,
"icon": Icons.person
},
{
"title": PageConst.shoppingOneRoute,
"color": Colors.green,
"icon": Icons.shopping_cart
},
{
"title": PageConst.dashboardOneRoute,
"color": Colors.red,
"icon": Icons.dashboard
},
{
"title": PageConst.timelineOneRoute,
"color": Colors.cyan,
"icon": Icons.timeline
},
{
"title": PageConst.settingsOneRoute,
"color": Colors.brown,
"icon": Icons.settings
},
];
@immutable
class CommonDrawer extends StatelessWidget {
CommonDrawer({this.context, this.title});
final String title;
final BuildContext context;
Widget _item(context, item) => ListTile(
title: Text(
item["title"].substring(1),
style: TextStyle(
color: Colors.grey[900],
fontWeight: FontWeight.w700,
fontSize: TEXT_NORMAL_SIZE),
),
leading: Icon(
item["icon"],
color: item["color"],
),
onTap: () {
var isEqual = item["title"].substring(1) == title;
if (isEqual || this.context == null) return;
var pageName = item["title"];
Navigator.pop(context);
Navigator.pop(this.context);
Navigator.pushNamed(context, pageName);
},
);
Widget _header() => UserAccountsDrawerHeader(
currentAccountPicture: CircleAvatar(
backgroundImage: AssetImage(ImagePath.nbImage),
),
accountName: Text(StringConst.DEVELOPER),
accountEmail: Text(StringConst.DEV_EMAIL),
);
@override
Widget build(BuildContext context) {
var list = List<Widget>();
list.add(_header());
for (var item in ITEMS) {
list.add(_item(context, item));
}
list.add(AboutMeTitle());
return Drawer(
child: ListView(
padding: EdgeInsets.zero,
children: list,
),
);
}
}
3. Other
we can the class Drawer above, but can write our widget instead of it, just the same as the other page;
The whole project is : https://github.com/nb312/flutter_ui_app
Let's see the render for our code.
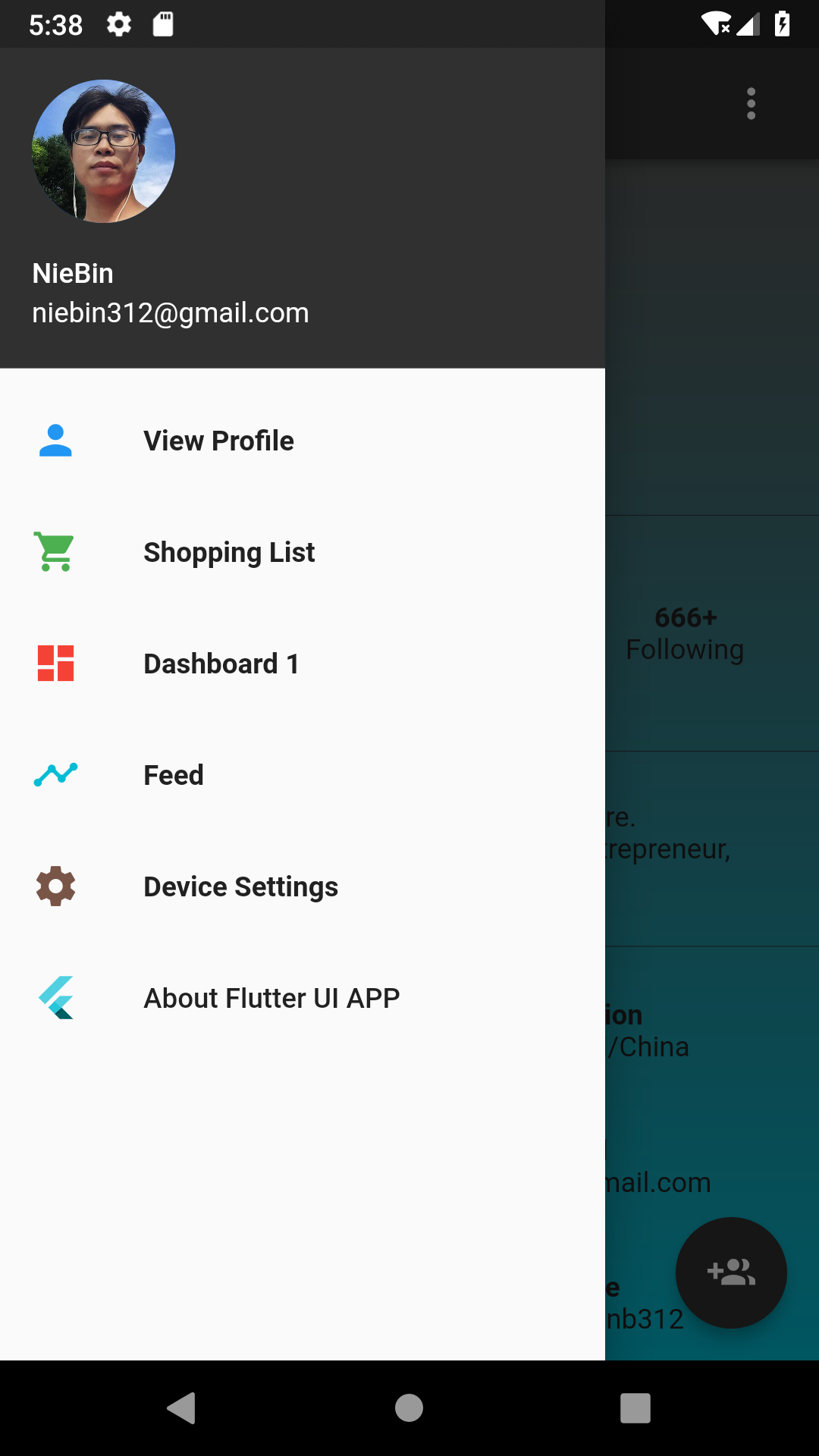