In this example, I'm going to share an example of how to select items in a ListView with a Trailing Icon and changing the style of App Bar.
Here is how the output of the program is going to look like. You can see the items are long pressed, the checkbox icons will appear and allow the user to select the items. When the back button in AppBar is pressed, everything is reset back to normal.
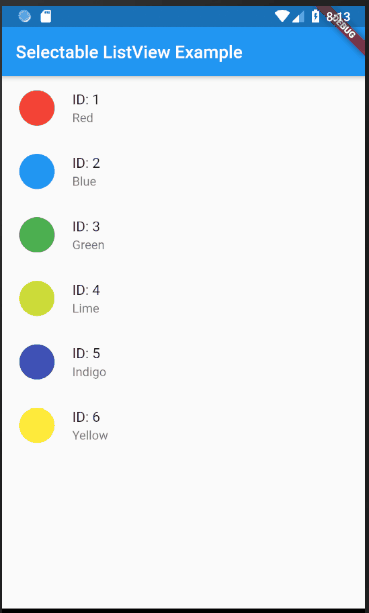
On LongPress of the ListView items, it will show the Trailing CheckBoxes which will allow user to Tap on the item and select the item.
By extending this example, you can actually keep track of the items selected or delete them. However, I'm not implementing this in this example.
Here is the full code from main.dart file. Copy-paste and run the program to see the above output.
import 'dart:developer';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
bool selectingmode = false;
List<Paint> paints = <Paint>[
Paint(1, 'Red', Colors.red),
Paint(2, 'Blue', Colors.blue),
Paint(3, 'Green', Colors.green),
Paint(4, 'Lime', Colors.lime),
Paint(5, 'Indigo', Colors.indigo),
Paint(6, 'Yellow', Colors.yellow)
];
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
leading: selectingmode
? IconButton(
icon: const Icon(Icons.arrow_back),
onPressed: () {
setState(() {
selectingmode = false;
paints.forEach((p) => p.selected = false);
});
},
)
: null,
title: Text("Selectable ListView Example"),
),
body: ListView(
children: List.generate(paints.length, (index) {
return ListTile(
onLongPress: () {
setState(() {
selectingmode = true;
});
},
onTap: () {
setState(() {
if (selectingmode) {
paints[index].selected = !paints[index].selected;
log(paints[index].selected.toString());
}
});
},
selected: paints[index].selected,
leading: GestureDetector(
behavior: HitTestBehavior.opaque,
onTap: () {},
child: Container(
width: 48,
height: 48,
padding: EdgeInsets.symmetric(vertical: 4.0),
alignment: Alignment.center,
child: CircleAvatar(
backgroundColor: paints[index].colorpicture,
),
),
),
title: Text('ID: ' + paints[index].id.toString()),
subtitle: Text(paints[index].title),
trailing: (selectingmode)
? ((paints[index].selected)
? Icon(Icons.check_box)
: Icon(Icons.check_box_outline_blank))
: null,
);
}),
),
),
);
}
}
class Paint {
final int id;
final String title;
final Color colorpicture;
bool selected = false;
Paint(this.id, this.title, this.colorpicture);
}
Hope this will be useful to you.
Thanks,Srikanth