Implementing a QR Code Scanner in flutter is pretty simple. We are going to use qrscan package in this tutorial to implement a QR Code scanner.
The package will also allow you to scan Barcodes.
Here is an illustration of what we will achieve with this tutorial.
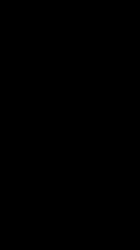
Let's begin by adding the package dependency inside our pubspec.yaml file
dependencies:
flutter:
sdk: flutter
qrscan: ^0.2.17
Next, use the following code in your main.dart file to get a QR Code scanner in your flutter app.
Here, the RaisedButton will trigger the scanQRCode() method. Inside this method, we will await the response generated by the scan() method which will be the output of the QR Code in the form of a string.
import 'package:flutter/material.dart';
import 'package:qrscan/qrscan.dart' as scanner;
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'QRCode Demo',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
String scanResult = '';
//function that launches the scanner
Future scanQRCode() async {
String cameraScanResult = await scanner.scan();
setState(() {
scanResult = cameraScanResult;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Scan Demo'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
scanResult == '' ? Text('Result will be displayed here') : Text(scanResult),
SizedBox(height: 20),
RaisedButton(
color: Colors.blue,
child: Text('Click To Scan', style: TextStyle(color: Colors.white),),
onPressed: scanQRCode,
)
],
),
),
);
}
}