In this example, I'm going to share the code on how to add an indicator to Bottomnavigationbar in flutter.
Here is the output of what you are going to achieve from this example.
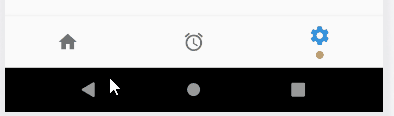
Here is the complete code from main.dart file. You can simply copy-paste and run to check the output.
import 'package:flutter/material.dart';
void main() {
runApp(App());
}
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(),
home: MainPage(),
);
}
}
class MainPage extends StatefulWidget {
@override
_MainPageState createState() => _MainPageState();
}
class _MainPageState extends State<MainPage> {
int _selectedIndex = 0;
void _onItemTappped(int value) {
setState(() {
_selectedIndex = value;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: [
BottomNavigationBarItem(
icon: Icon(Icons.home),
title: showIndicator(_selectedIndex == 0)),
BottomNavigationBarItem(
icon: Icon(Icons.access_alarm),
title: showIndicator(_selectedIndex == 1)),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
title: showIndicator(_selectedIndex == 2)),
],
onTap: _onItemTappped,
currentIndex: _selectedIndex,
),
);
}
}
Widget showIndicator(bool show) {
return show
? Padding(
padding: const EdgeInsets.only(top: 4),
child: Icon(Icons.brightness_1, size: 10, color: Colors.orange),
)
: SizedBox();
}
Hope this post is useful to you.
Thanks,
Srikanth