In this article, I'm going to show you how to create a waveclipper in flutter.
Below is what we are going to design for this example.
In this program, we are using ClipPath widget to clips its child (which is a container in this program) using a path.
The path is specified in the below class.
class BottomWaveClipper extends CustomClipper<Path> { @override Path getClip(Size size) { var path = new Path(); path.lineTo(0.0, size.height - 40); path.quadraticBezierTo( size.width / 4, size.height - 80, size.width / 2, size.height - 40); path.quadraticBezierTo(size.width - (size.width / 4), size.height, size.width, size.height - 40); path.lineTo(size.width, 0.0); path.close(); return path; } @override bool shouldReclip(CustomClipper<Path> oldClipper) { return false; } }
quadratickBezierTo method adds a quadratic bezier segment that curves from the current point to the given point (x2,y2), using the control point (x1,y1). Below is the signature of the method,
void quadraticBezierTo (
double x1,
double y1,
double x2,
double y2
)
Below is how the values provided will reflect in the final curve. You can draw is upside down, by modifying the x1, y1.
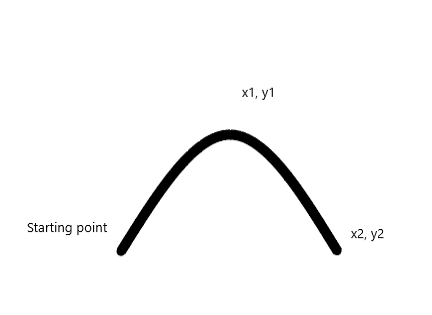
The path.lineTo method adds a straight line segment from the current point to the given point. Below is the signature of the method,
void lineTo (
double x,
double y
)
Here is the full code from main.dart file, you can simply copy-paste and run to check this example.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
double padValue = 1;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("BottomWaveClipper"),
),
body: Center(
child: MyClipPath(),
),
),
);
}
}
class MyClipPath extends StatelessWidget {
final Color backgroundColor = Colors.red;
@override
Widget build(BuildContext context) {
return ClipPath(
clipper: BottomWaveClipper(),
child: Container(
color: backgroundColor,
width: 300,
height: 200,
),
);
}
}
class BottomWaveClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
var path = new Path();
path.lineTo(0.0, size.height - 40);
path.quadraticBezierTo(
size.width / 4, size.height - 80, size.width / 2, size.height - 40);
path.quadraticBezierTo(size.width - (size.width / 4), size.height,
size.width, size.height - 40);
path.lineTo(size.width, 0.0);
path.close();
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return false;
}
}