In this article, I'm going to share code that will help you to scale in and out widgets in flutter using the ScaleTransition widget.
This is how the output of this example is going to look like.
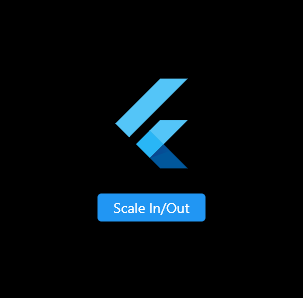
Here is the complete code from main.dart files. You can simply copy-paste the code to try this example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MyPage(),
);
}
}
class MyPage extends StatelessWidget {
const MyPage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MyAnimation();
}
}
class MyAnimation extends StatefulWidget {
const MyAnimation({Key? key}) : super(key: key);
@override
_MyAnimationState createState() => _MyAnimationState();
}
class _MyAnimationState extends State<MyAnimation>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
double value = 1.0;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: 500), vsync: this);
_animation = Tween(
begin: 1.0,
end: 0.0,
).animate(CurvedAnimation(parent: _controller, curve: Curves.easeIn));
}
@override
void dispose() {
super.dispose();
_controller.dispose();
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ScaleTransition(
scale: _animation,
child: const FlutterLogo(
size: 100.0,
),
),
const SizedBox(
height: 20.0,
),
ElevatedButton(
onPressed: () {
setState(() {
value = value == 1 ? 0 : 1;
if (value == 0) {
_controller.animateTo(1.0);
} else {
_controller.animateBack(0.0);
}
});
},
child: const Text('Scale In/Out'))
],
);
}
}
Hope this example is useful to you.Thanks,
Sri