<p>In this post, I'm going to show you how to show a Dialog box on a Button Click. The final output is going to look like below. On button click, you will see the Dialog box shown below.</p> <p>);</p> <p>class MyApp extends StatelessWidget { // This widget is the root of your application.</p> <p>@override Widget build(BuildContext context) { return new MaterialApp( title: 'Flutter Demo', theme: new ThemeData( primarySwatch: Colors.blue, ), home: new MyHomePage(title: 'Flutter Demo Home Page'), ); } }</p> <p>class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key);</p> <p>final String title;</p> <p>@override _MyHomePageState createState() => new _MyHomePageState(); }</p> <p>class _MyHomePageState extends State { void _showcontent() { showDialog( context: context, barrierDismissible: false, // user must tap button!</p> <pre> builder: (BuildContext context) { return new AlertDialog( title: new Text('You clicked on'), content: new SingleChildScrollView( child: new ListBody( children: [ new Text('This is a Dialog Box. Click OK to Close.'), ], ), ), actions: [ new FlatButton( child: new Text('Ok'), onPressed: () { Navigator.of(context).pop(); }, ), ], ); }, ); </pre> <p>}</p> <p>@override Widget build(BuildContext context) { return new Scaffold( appBar: new AppBar( title: new Text(widget.title), ), body: new Center( child: new Column( mainAxisAlignment: MainAxisAlignment.center, children: [ new Text( 'Push the button to show a Dialog:', ), new RaisedButton( onPressed: _showcontent, color: Colors.blueAccent, child: new Text('Click here', style: TextStyle(color: Colors.white)), ), ], ), ), ); } }</p> <p>Let me know if this helps through your comments.</p> <p>Thanks, Srikanth</p>
How to show a Dialog Box on a Button Click in Flutter?
This Article is posted by seven.srikanth at 9/29/2018 4:16:27 AM
Check out our other latest articles
Safearea widget - How to avoid visual overlap with Notch on flutter mobile app?How to convert row of widgets into column of widgets in flutter based on screen size?
How to run Flutter programs from GitHub?
How to get screen orientation in flutter?
NavigationRail example in flutter
Tags: show a Dialog Box on a Button Click; Popup Dialog Box; Button Click Event; Coloring Button; AlertDialog Widget;
Check out our other latest articles
Safearea widget - How to avoid visual overlap with Notch on flutter mobile app?How to convert row of widgets into column of widgets in flutter based on screen size?
How to run Flutter programs from GitHub?
How to get screen orientation in flutter?
NavigationRail example in flutter
1 Comments
Login to comment.
Recent Comments
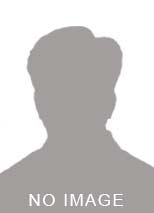
ice.simx at 6/19/2019
Code Correction; instead of ShowDialog<Null> it should be ShowDialog<void> https://api.flutter.dev/flutter/material/AlertDialog-class.html
Login to Reply.