<p>In this example, I've implemented Flutter GridView Widget into a TabBar. Each tab will have different GirdView layout implementations. Various Grid layouts are GirdView, GridView.count, GridView.builder, GridView.custom, GridView.extent.</p> <p>As per Flutter documentation, the most commonly used grid layouts are GridView.count, which creates a layout with a fixed number of tiles in the cross axis, and GridView.extent, which creates a layout with tiles that have a maximum cross-axis extent. A custom SliverGridDelegate can produce an arbitrary 2D arrangement of children, including arrangements that are unaligned or overlapping. If you are a beginner and want to try a simple example, then check this out. <a href="https://fluttercentral.com/Articles/Post/31">https://fluttercentral.com/Articles/Post/31font-size: 1rem;</a></p> <p>To create a grid with a large (or infinite) number of children, use the GridView.builder constructor with either a SliverGridDelegateWithFixedCrossAxisCount or a SliverGridDelegateWithMaxCrossAxisExtent for the gridDelegate. To use a custom SliverChildDelegate, use GridView.custom.</p> <p>Here is the final output of this program.</p> <p> { // TODO: implement build return MaterialApp( home: DefaultTabController( length: 5, child: Scaffold( appBar: AppBar( title: Text('Grid Demo'), bottom: TabBar( tabs: [ Tab( text: 'GridView', ), Tab( text: 'GridView.Count', ), Tab( text: 'GridView.builder', ), Tab( text: 'GridView.custom', ), Tab( text: 'GridView.extent', ), ], isScrollable: true, )), body: TabBarView(children: [ GridView( scrollDirection: Axis.vertical, controller: ScrollController(), gridDelegate: SliverGridDelegateWithMaxCrossAxisExtent( maxCrossAxisExtent: 200.0), children: List.generate(100, (index) { return Container( padding: EdgeInsets.all(20.0), child: Center( child: GridTile( footer: Text( 'Item $index', textAlign: TextAlign.center, ), header: Text( 'SubItem $index', textAlign: TextAlign.center, ), child: Icon(Icons.access_alarm, size: 40.0, color: Colors.white30), ), ), color: Colors.blue[400], margin: EdgeInsets.all(1.0), ); }), ), GridView.count( crossAxisCount: 2, children: List.generate(100, (index) { return Container( padding: EdgeInsets.all(20.0), child: Center( child: GridTile( footer: Text( 'Item $index', textAlign: TextAlign.center, ), header: Text( 'SubItem $index', textAlign: TextAlign.center, ), child: Icon(Icons.access_alarm, size: 40.0, color: Colors.white30), ), ), color: Colors.blue[400], margin: EdgeInsets.all(1.0), ); })), GridView.builder( itemCount: 50, gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 3), itemBuilder: (BuildContext context, int index) { //if (index</p> <p>Let me know your queries through comments. Thanks, Srikanth</p>
GridView Widget - Grid layouts Implementation - GirdView, GridView.count, GridView.builder, GridView.custom, GridView.extent
This Article is posted by seven.srikanth at 10/2/2018 11:30:09 AM
Check out our other latest articles
Safearea widget - How to avoid visual overlap with Notch on flutter mobile app?How to convert row of widgets into column of widgets in flutter based on screen size?
How to run Flutter programs from GitHub?
How to get screen orientation in flutter?
NavigationRail example in flutter
Tags: GridView Widget - Grid layouts Implementation - GirdView, GridView.count, GridView.builder, GridView.custom, GridView.extent ; How to implement GridView in Flutter? GridView Widget; GridView with Images;
Check out our other latest articles
Safearea widget - How to avoid visual overlap with Notch on flutter mobile app?How to convert row of widgets into column of widgets in flutter based on screen size?
How to run Flutter programs from GitHub?
How to get screen orientation in flutter?
NavigationRail example in flutter
3 Comments
Recent Comments
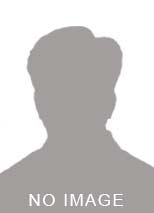
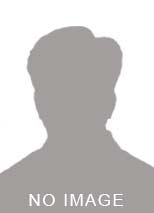
abhisonawane126 at 10/21/2019
Thanks for the great example. How to get the index value of elements in gridView , i need to animate the elements in grid view ... ?
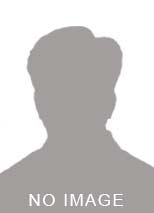
seven.srikanth at 11/6/2019
Thanks for your comment. $index has the index value. Please let me know if that answers your question.