Here is an example of how to open a page on top of another page without hiding the Drawer.
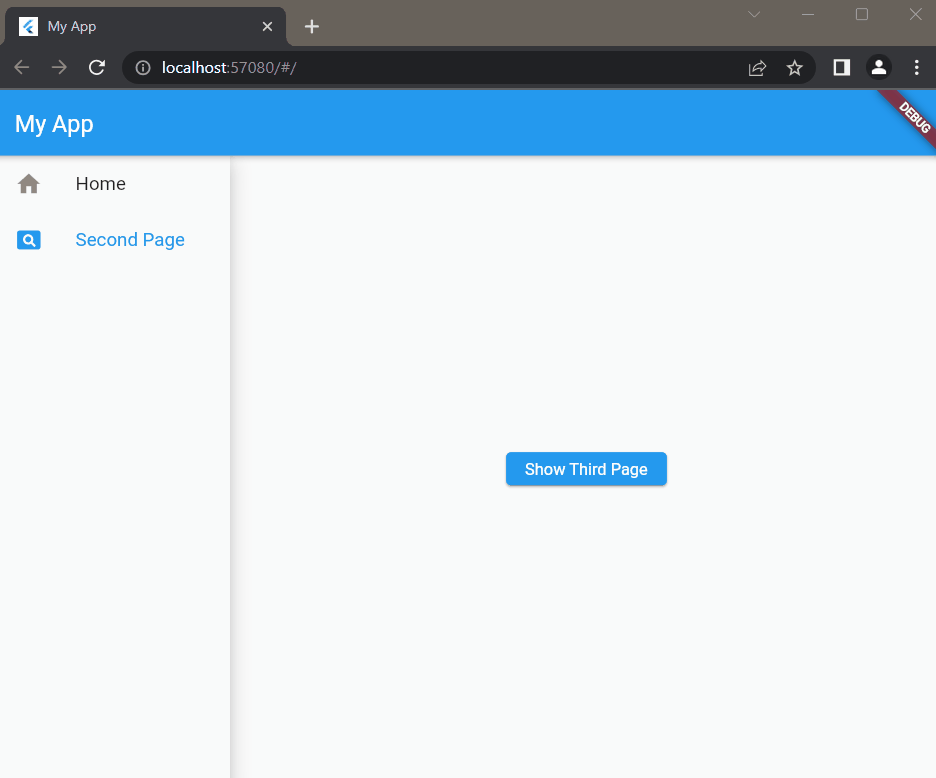
Here is the complete code for the above example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key
? key}) : super(key
: key);
@
override
Widget build(BuildContext context) {
return MaterialApp(
title
: 'My App',
theme
: ThemeData(
primarySwatch
: Colors.blue,
),
home
: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key
? key}) : super(key
: key);
@
override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
int _currentIndex = 0;
OverlayEntry
? _overlayEntry;
final List<Widget> _pages = const [
HomePageContent(),
SecondPageContent(),
];
@
override
Widget build(BuildContext context) {
return Scaffold(
appBar
: AppBar(
title
: const Text('My App'),
),
body
: Row(
children
: [
SizedBox(
width
: 200, // Adjust the width to fit your design
child
: Drawer(
child
: NavigationDrawer(
currentIndex
: _currentIndex,
onItemSelected
: (index) {
setState(() {
_currentIndex = index;
});
// Navigator.pop(context); // Close the drawer
},
),
),
),
Expanded(
child
: Stack(
children
: [
Positioned.fill(
child
: _pages[_currentIndex],
),
if (_currentIndex == 1 && _overlayEntry == null)
GestureDetector(
onTap
: () {
_showThirdPageOverlay(context);
},
child
: IgnorePointer(
child
: _pages[_currentIndex],
),
),
],
),
),
],
),
);
}
void _showThirdPageOverlay(BuildContext context) {
if (_overlayEntry == null) {
final RenderBox renderBox = context.findRenderObject() as RenderBox;
final size = renderBox.size;
final offset = renderBox.localToGlobal(Offset.zero);
final topBoundary = offset.dy;
final bottomBoundary = offset.dy + size.height;
_overlayEntry = OverlayEntry(
builder
: (context) {
return Positioned(
top
: topBoundary,
bottom
: MediaQuery.of(context).size.height - bottomBoundary,
left
: offset.dx,
right
: MediaQuery.of(context).size.width - offset.dx - size.width,
child
: thirdpagewidget(),
);
},
);
Overlay.of(context).insert(_overlayEntry
!);
}
}
void _hideThirdPageOverlay() {
_overlayEntry
?.remove();
_overlayEntry = null;
}
thirdpagewidget(){
return WillPopScope(
onWillPop
: () async {
_hideThirdPageOverlay();
return false;
},
child
: Scaffold(
appBar
: AppBar(
title
: const Text('Third Page'),
actions
: [
IconButton(
icon
: const Icon(Icons.close),
onPressed
: () {
_hideThirdPageOverlay();
},
),
],
),
body
: GestureDetector(
onTap
: () {
_hideThirdPageOverlay();
},
child
: Container(
color
: Colors.yellow,
child
: const Center(
child
: Text('Third Page'),
),
),
),
),
);
}
}
class NavigationDrawer extends StatelessWidget {
final int currentIndex;
final Function(int) onItemSelected;
const NavigationDrawer({
required this.currentIndex,
required this.onItemSelected,
Key
? key,
}) : super(key
: key);
@
override
Widget build(BuildContext context) {
return ListView(
padding
: EdgeInsets.zero,
children
: [
ListTile(
leading
: const Icon(Icons.home),
title
: const Text('Home'),
onTap
: () => onItemSelected(0),
selected
: currentIndex == 0,
),
ListTile(
leading
: const Icon(Icons.pageview),
title
: const Text('Second Page'),
onTap
: () => onItemSelected(1),
selected
: currentIndex == 1,
),
],
);
}
}
class HomePageContent extends StatelessWidget {
const HomePageContent({Key
? key}) : super(key
: key);
@
override
Widget build(BuildContext context) {
return const Center(
child
: Text('Home Page'),
);
}
}
class SecondPageContent extends StatelessWidget {
const SecondPageContent({Key
? key}) : super(key
: key);
@
override
Widget build(BuildContext context) {
return Center(
child
: ElevatedButton(
child
: const Text('Show Third Page'),
onPressed
: () {
_HomePageState homePageState =
context.findAncestorStateOfType<_HomePageState>()!;
homePageState._showThirdPageOverlay(context);
},
),
);
}
}
Thanks,Srikanth