Buttons are the basic UI component of any framework. In this tutorial, we will do a Flutter Raised Button Example. Flutter’s basic motto is to create stunning UI. There are 7 different types of buttons in Flutter. Here you can learn about all those flutter’s buttons.
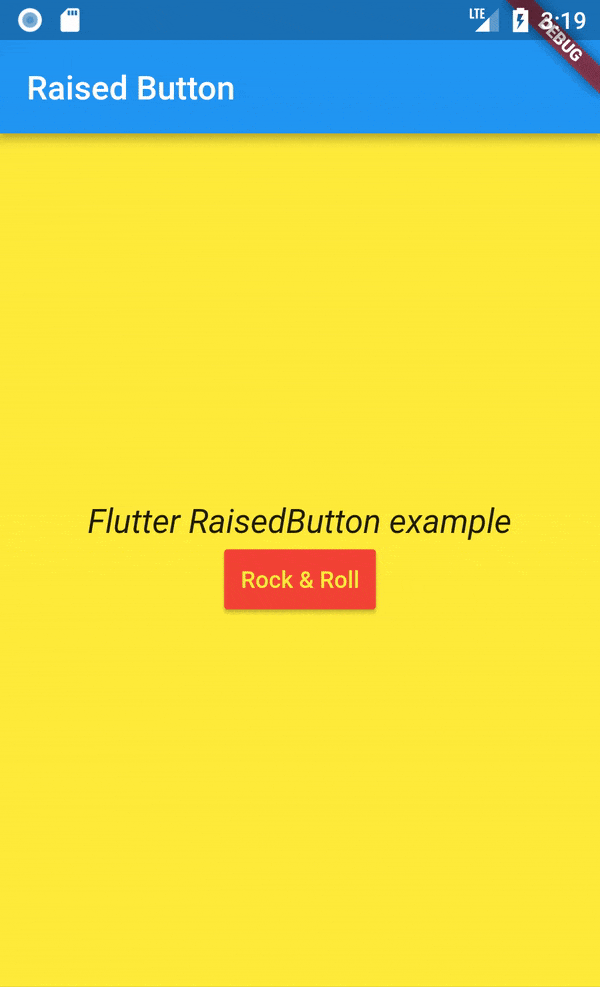
Code :
RaisedButton(child: Text("Rock & Roll"),
onPressed: _changeText,
color: Colors.red,
textColor: Colors.yellow,
padding: EdgeInsets.fromLTRB(10, 10, 10, 10),
splashColor: Colors.grey,
)
RAISEDBUTTON PROPERTIES
Raised button has a single constructor but a long list of attributes. I am just mentioning the basic one we have used.
- onPressed: onPressed function is called when we click on the button.
- color: Color set the button
color . Our buttoncolor is red. - textColor: This attribute is used to give the
color to thebutton’s text. - padding: Padding to the text inside the button.
- splash color: A
color effect that we get when we click on the button.
FLUTTER RAISED BUTTON EXAMPLE CODE :
void main() => runApp(MaterialApp(title: "RaisedButton", home: MainActivity()));
class MainActivity extends StatefulWidget {
@override
_MainActivityState createState() => _MainActivityState();
}
class _MainActivityState extends State {
String msg = 'Flutter RaisedButton example';
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.yellow,
appBar: AppBar(
title: Text('Raised Button'),
),
body: Container(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
msg,
style: TextStyle(fontSize: 20, fontStyle: FontStyle.italic),
),
RaisedButton(
child: Text("Rock & Roll"),
onPressed: _changeText,
color: Colors.red,
textColor: Colors.yellow,
padding: EdgeInsets.fromLTRB(10, 10, 10, 10),
splashColor: Colors.grey,
)
],
),
),
),
);
}
_changeText() {
setState(() {
if (msg.startsWith('F')) {
msg = 'I have learned FlutterRaised example ';
} else {
msg = 'Flutter RaisedButton example';
}
});
}
}
__________________________________________________________________________________
For More Info About Flutter :
Check Out Live Flutter Web Demo: http://nikhil.cf/