A drop-down button creates a nice overlay on the screen providing multiple options to choose from. It is fairly simple to implement a drop down box or drop down button in flutter, thanks to the amazing flutter team.
Flutter provides an out of the box widget, DropdownButton, to create a drop down button which can be placed anywhere in your app just like any other button.
Here is a quick illustration of a drop down button in flutter:
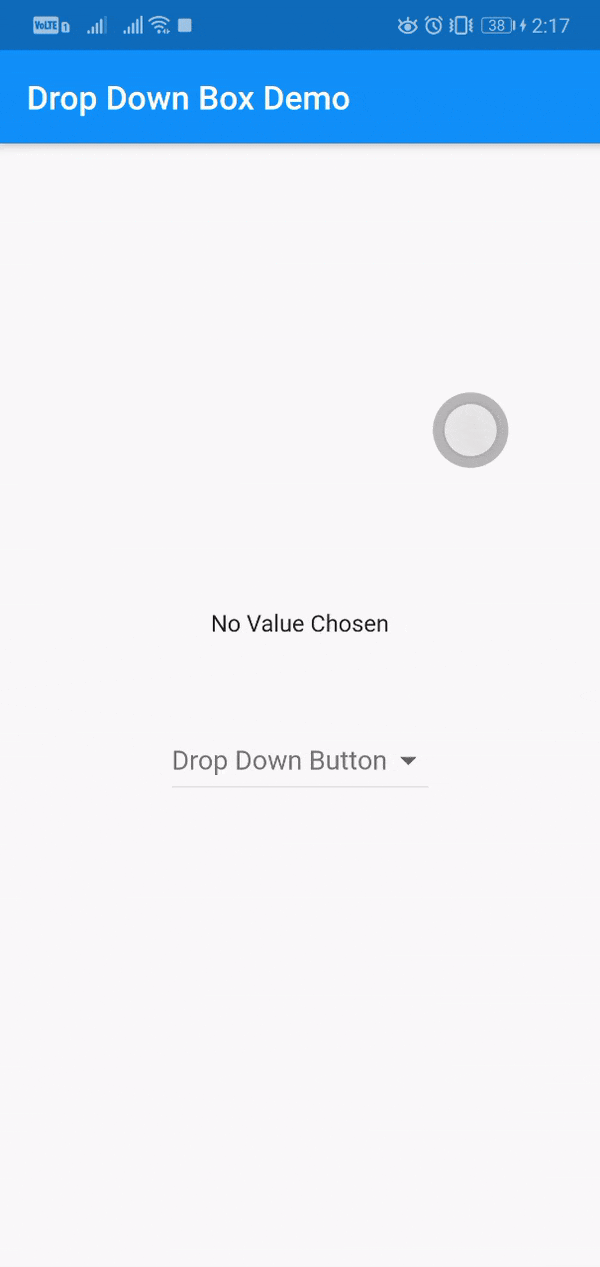
Key Aspects:
1. The DropdownButton widget takes a List of type DropdownMenuItem. In the above illustration, we have 4 DropdownMenuItem widgets.
2. DropdownMenuItem is one single item in the overlay/drop-down menu. It takes a value and a child widget as arguments. The child widget contains the actual widget that is displayed as an item of drop down. On the other hand, value contains the underlying value that the button generates.
3. Finally, onChanged property of the DropdownButton takes a function that triggers every time a value is selected or changed.
The following code snippet will add a drop down button similar to the one shown in the illustration.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Drop down Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Drop Down Box Demo'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String _dropDownButtonValue =
'No Value Chosen'; //initial value that is displayed before any option is chosen from drop down
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(widget.title), actions: <Widget>[]),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
_dropDownButtonValue,
),
SizedBox(
height: 50,
),
DropdownButton(
focusColor: Colors.blue,
hint: Text('Drop Down Button'), //text shown on the button (optional)
elevation: 3,
items: <String>['Dart', 'Python', 'Javascript', 'C++']
.map((String val) => DropdownMenuItem<String>(
value: val,
child: Text(val),
))
.toList(),
onChanged: (value) {
setState(() {
_dropDownButtonValue = value;
});
},
)
],
),
),
);
}
}
Here, the List of Strings map each String value to a DropdownMenuItem.
That was all for a drop down button in flutter.
Thank You!
Keep fluttering :)