When it comes to providing better user experience to users on mobile apps, making effective use of space is a must. Carousels come into play here by allowing the developers to show multiple items in a single, coveted space.
Thankfully, implementing carousels in flutter is pretty straightforward and all we need is the carousel_slider package.
By the end of this tutorial, you will be able to create a similar carousel in flutter.
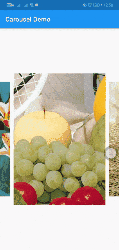
With no further ado, let's add the package dependency to our pubspec.yaml file
dependencies:
flutter:
sdk: flutter
carousel_slider: ^1.3.0
Next, use the following code snippet in your main.dart file to get the above illustration.
import 'package:flutter/material.dart';
import 'package:carousel_slider/carousel_slider.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Carousel Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Carousel Demo'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
List<String> imageLinks = [
'https://homepages.cae.wisc.edu/~ece533/images/fruits.png',
'https://homepages.cae.wisc.edu/~ece533/images/cat.png',
'https://homepages.cae.wisc.edu/~ece533/images/peppers.png',
'https://homepages.cae.wisc.edu/~ece533/images/tulips.png'
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
CarouselSlider(
height: 400.0,
items: imageLinks.map((imageLink) {
return Builder(
builder: (BuildContext context) {
return Container(
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.symmetric(horizontal: 5.0),
child: Image.network(
imageLink,
fit: BoxFit.cover,
));
},
);
}).toList(),
reverse:
false, //is false by default (reverses the order of items)
enableInfiniteScroll:
true, //is true by default (it scrolls back to item 1 after the last item)
autoPlay: true, //is false by default
initialPage:
0, //allows you to set the first item to be displayed
scrollDirection: Axis.horizontal, //can be set to Axis.vertical
pauseAutoPlayOnTouch: Duration(
seconds: 5), //it pauses the sliding if carousel is touched,
onPageChanged: (int pageNumber) {
//this triggers everytime a slide changes
},
viewportFraction: 0.8,
enlargeCenterPage: true, //is false by default
aspectRatio:
16 / 9, //if height is not specified, then this value is used
)
]
)
)
);
}
}
Brief Overview:
CarouselSlider widget takes has items property which takes a list of items to be displayed in the carousel. For efficient loading and memory saving, you can use a Map & Builder widget as shown in the above example to return a custom widget for every list item.