Flutter supports opening and viewing PDF files inside your apps through URLs, assets & local storage.
In this tutorial, we are going to see how we can launch pdf files in a flutter app using a package called
flutter_plugin_pdf_viewer.
This package can also be used to load & view pdf files from the local storage.
Let's have a look at the illustration before we dive into the code.
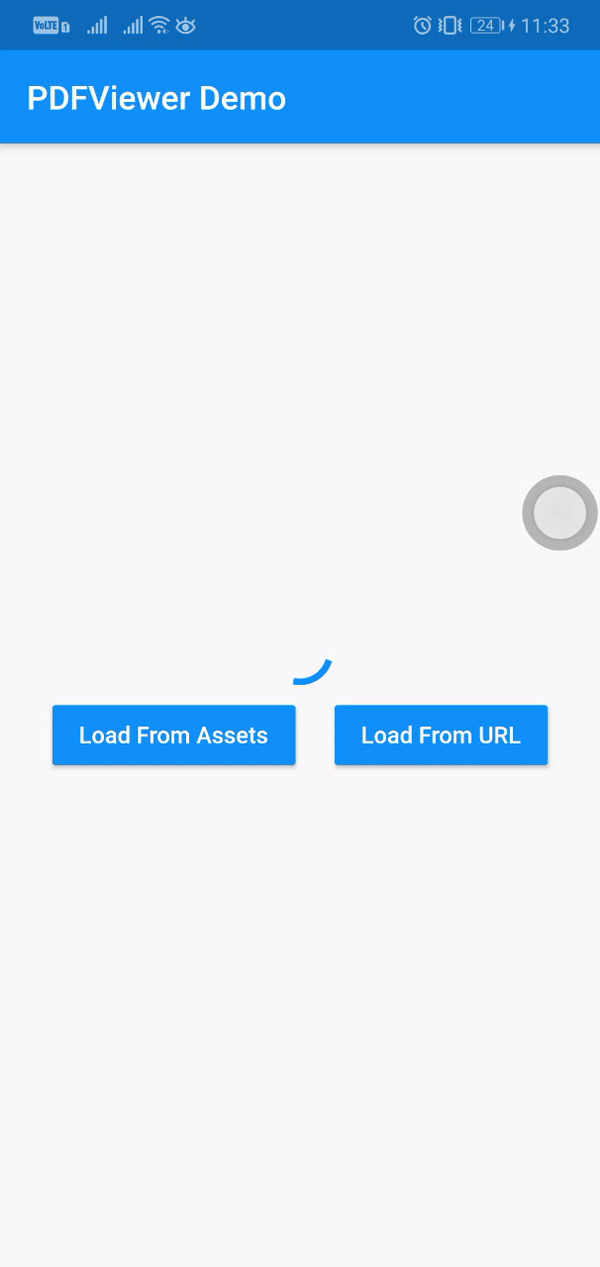
STEPS TO FOLLOW
- To begin with, add the package dependency as follows in your pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
flutter_plugin_pdf_viewer: ^1.0.7
- Create a new folder named assets and add a pdf file to this folder. The file should be named "sample.pdf
- Add the assets path to the pubspec.yaml file.
# To add assets to your application, add an assets section, like this:
assets:
- assets/sample.pdf
- Paste the following code inside your main.dart file. An explanation for the same is provided below the code.
import 'package:flutter/material.dart'; import 'package:flutter_plugin_pdf_viewer/flutter_plugin_pdf_viewer.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'PDFViewer Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(title: 'PDFViewer Demo'), ); } } class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { bool _isLoading = true; PDFDocument doc; void _loadFromAssets() async { setState(() { _isLoading = true; }); doc = await PDFDocument.fromAsset('assets/sample.pdf'); setState(() { _isLoading = false; }); } void _loadFromUrl() async { setState(() { _isLoading = true; }); doc = await PDFDocument.fromURL( 'https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf'); setState(() { _isLoading = false; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Flexible( flex: 8, child: _isLoading ? CircularProgressIndicator() : PDFViewer( document: doc, ), ), Flexible( flex: 2, child: Padding( padding: const EdgeInsets.all(8.0), child: Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ RaisedButton( color: Colors.blue, child: Text( 'Load From Assets', style: TextStyle(color: Colors.white), ), onPressed: _loadFromAssets, ), RaisedButton( color: Colors.blue, child: Text( 'Load From URL', style: TextStyle(color: Colors.white), ), onPressed: _loadFromUrl, ), ], ), ), ), ], ), ), ); } }
Code Overview:
We add two RaisedButton which are responsible for triggering two different methods _loadFromAssets & _loadFromUrl.
Each of these methods updates the value fo doc variable of type PDFDocument which is actually rendered to the screen of the app.
Do comment below if you have any doubts or questions.
Happy Fluttering :)