In this tutorial, we are going to have a look at adding or displaying an image from stored on the device without adding it to assets.
For this purpose, we are going to make use of the file_picker package.
Let's have a look at the tiny illustration of how it looks before writing the code.
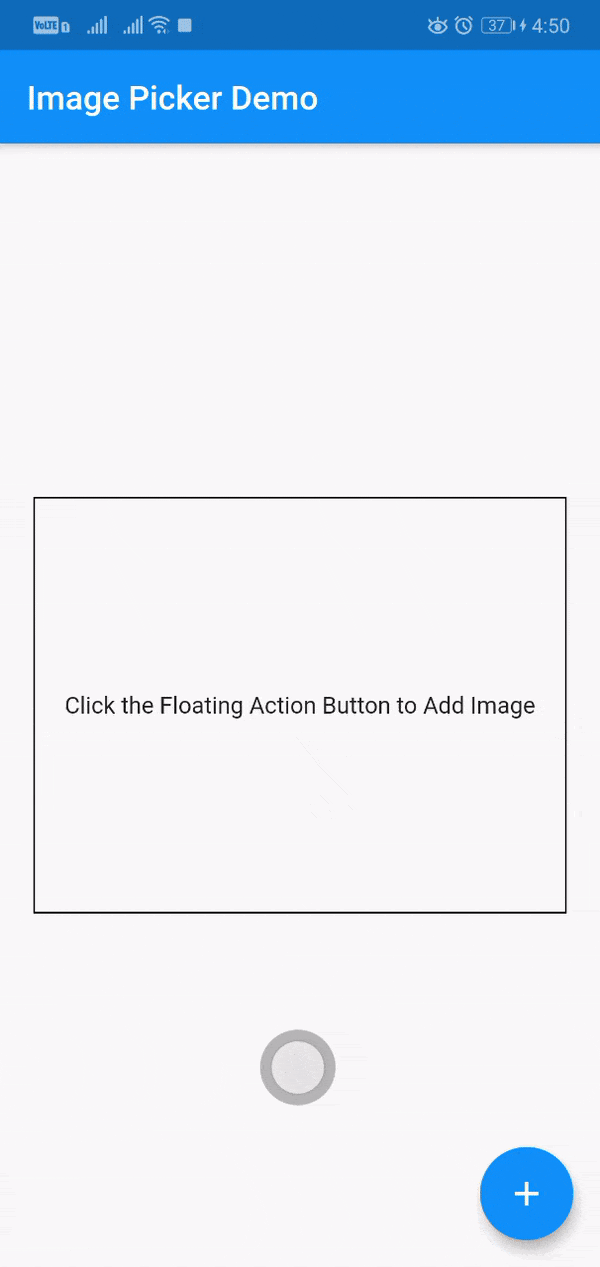
STEPS TO FOLLOW:
1. Add the package dependency to your pubspec.yaml file as follows
dependencies:
flutter:
sdk: flutter
file_picker: ^1.5.0+2
2. Use the following code inside your main.dart file.
import 'dart:io'; import 'package:file_picker/file_picker.dart'; import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Image Picker Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(title: 'Image Picker Demo'), debugShowCheckedModeBanner: false, ); } } class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { File imageFile; _loadImage() async { File file = await FilePicker.getFile(); setState(() { imageFile = file; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( height: 250, width: 320, decoration: BoxDecoration( border: Border.all( width: 1, ), ), margin: EdgeInsets.all(16.0), child: imageFile == null ? Center( child: Text( 'Click the Floating Action Button to Add Image', textAlign: TextAlign.center, ), ) : Image.file( imageFile, fit: BoxFit.contain, )) ], ), ), floatingActionButton: FloatingActionButton( onPressed: _loadImage, tooltip: 'Load Image', child: Icon(Icons.add), ), ); } }
Quick Overview:
- FilePicker package offers an asynchronous method getFile() which returns the file selected from the local storage.
- You can also restrict the files loaded on the basis of file types and file extensions by providing them as arguments inside the getFile() method.
- It also offers other methods such as getFilePath() which returns the path of the file stored locally.
- Alongside, it also has methods to select multiple files at once namely getMultiFile() and getMultipleFilePath().
If you have any questions, do ask them in the comments section.
Keep Fluttering :)