Usually, when you add a container inside another container, the boundaries are not restricted. So, let's find out how to restrict its boundaries by adding a container inside another container.
Here is an example of what you are going to achieve in this example.
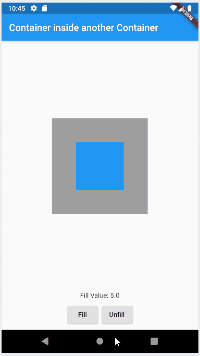
To achieve this, we are using a ClipRRect class. It's a widget that clips its child using a rounder rectangle.
Here is full code from main.dart file. All you need to do is, copy-paste and run to see the output.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { double padValue = 1; @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text("Container inside another Container"), ), body: Column( children: [ Expanded( child: Center( child: MyFillingContainer( progress: padValue / 10, size: 200, backgroundColor: Colors.grey, progressColor: Colors.blue, ), ), ), Text('Fill Value: $padValue'), ButtonBar( alignment: MainAxisAlignment.center, children: [ RaisedButton( child: Text('Fill'), onPressed: () { setState(() { padValue = padValue + 1; }); }, ), RaisedButton( child: Text('Unfill'), onPressed: () { setState(() { if (padValue != 0) { padValue = padValue - 1; } }); }, ) ], ), ], ), ), ); } } class MyFillingContainer extends StatelessWidget { final double progress; final double size; final Color backgroundColor; final Color progressColor; const MyFillingContainer( {Key key, this.progress, this.size, this.backgroundColor, this.progressColor}) : super(key: key); @override Widget build(BuildContext context) { return ClipRRect( child: SizedBox( height: size, width: size, child: Stack( children: [ Container( color: backgroundColor, ), Center( child: Container( height: size * progress, width: size * progress, color: progressColor, ), ) ]), ), ); } }
Thanks,
Srikanth